如何在添加圖像時(shí)自動選擇背景?
有沒有想過 Instagram 如何在你添加圖像時(shí)自動選擇背景?!
他們通過不同的算法分析你的圖片并生成與圖像匹配的背景。他們主要使用圖像中存在的“顏色”來處理輸出。
在本文中,你可以找到 2 種技術(shù)來檢測輸入圖像的合適背景。在最終用戶產(chǎn)品中使用這些算法時(shí),這些方法有點(diǎn)幼稚,但對于學(xué)習(xí)新東西的開發(fā)人員來說,這些方法非常方便且易于復(fù)制。
讓我們了解第一種方法
在這種情況下,我們只需將RGB矩陣分離為單獨(dú)的顏色通道,然后使用Counter() 函數(shù)分別對3個(gè)RGB矩陣中的每個(gè)像素值進(jìn)行頻率計(jì)數(shù)。
然后,選擇10個(gè)出現(xiàn)頻率最高的值并取它們的平均值來獲得結(jié)果像素值。
最后,只需使用np.zeros() 生成一個(gè)空白圖像,并用獲得的背景色填充它即可顯示最終結(jié)果。這種技術(shù)只需40行代碼就可以產(chǎn)生結(jié)果!
以下是第一種方法的完整代碼:
import cv2
import numpy as np
from collections import Counter
file_path='YOUR_FILE_PATH'
def find_background(path=None):
if(path is not None):
img=cv2.imread(path)
img=cv2.resize(img,(800,600))
blue,green,red=cv2.split(img)
blue=blue.flatten()
green=green.flatten()
red=red.flatten()
blue_counter=Counter(blue)
green_counter=Counter(green)
red_counter=Counter(red)
blue_most=blue_counter.most_common(10)
blue_avg=[i for i,j in blue_most]
blue_avg=int(np.mean(blue_avg)
green_most=green_counter.most_common(10)
green_avg=[i for i,j in green_most]
green_avg=int(np.mean(green_avg))
red_most=red_counter.most_common(10)
red_avg=[i for i,j in red_most]
red_avg=int(np.mean(red_avg))
background=[blue_avg,green_avg,red_avg]
bg=np.zeros((512,512,3),np.uint8)
bg_color=cv2.rectangle(bg,(0,0),(512,512),background,-1)
return(bg_color)
else:
return(None)
bg_image=find_background(file_path)
cv2.imshow('Image',img)
cv2.imshow('Background',bg_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
我們得到的原始圖像和由此產(chǎn)生的背景顏色
獲得的原始圖像和由此產(chǎn)生的背景顏色
什么是更復(fù)雜的方法來做到這一點(diǎn)?“ML”在哪里?!
實(shí)現(xiàn)相同目標(biāo)的另一種方法是使用機(jī)器學(xué)習(xí)算法為我們提供我們想要計(jì)算最頻繁的顏色分離。
第二種方法對 RGB 值使用 K-Means 聚類算法,并找出圖像中存在的一組不同顏色的聚類。之后,再次利用頻率計(jì)數(shù),最后找到背景色。這種方法涉及到無監(jiān)督機(jī)器學(xué)習(xí)的使用,其應(yīng)用范圍遠(yuǎn)遠(yuǎn)超出了背景顏色檢測。
圖像分割任務(wù)大量使用這種方法對圖像進(jìn)行K均值聚類。
以下是K-Means方法的完整代碼:
import cv2
import numpy as np
from collections import Counter
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
img=cv2.imread('YOUR_FILE_NAME')
img=cv2.resize(img,(500,500))
plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
#Reshaping into a R,G,B -(2x3) 2D-Matrix
modified_img=img.reshape(img.shape[0]*img.shape[1],3)
#print(modified_img)
#plotting all the points in 3D space.
x=modified_img[:,0]
y=modified_img[:,1]
z=modified_img[:,2]
fig = plt.figure(figsize=(5,5))
ax = plt.a(chǎn)xes(projection ='3d')
ax.scatter(x, y, z)
plt.show()
# Performing unpsupervised learning using K-Means
kmean=KMeans(n_clusters=10)
labels=kmean.fit_predict(modified_img)
#print(kmean.labels_)
#Points as shown according to the labels of KMeans
x=modified_img[:,0]
y=modified_img[:,1]
z=modified_img[:,2]
fig = plt.figure(figsize=(7, 7))
ax = plt.a(chǎn)xes(projection ='3d')
ax.scatter(x, y, z, c=kmean.labels_, cmap='rainbow')
ax.scatter(kmean.cluster_centers_[:,0] ,kmean.cluster_centers_[:,1],kmean.cluster_centers_[:,2], color='black')
plt.show()
#Making frequency of each label set i.e count of no. of data-points in each label
count=Counter(labels)
center_color=kmean.cluster_centers_
ordered_color=[center_color[i] for i in count.keys()]
def rgb2hex(color):
return "#{:02x}{:02x}{:02x}".format(int(color[0]),
int(color[1]), int(color[2]))
hex_color=[rgb2hex(ordered_color[i]) for i in count.keys()]
plt.figure(figsize = (8, 6))
plt.pie(count.values(),labels=hex_color,colors=hex_color,shadow=True)
def get_key(val):
for key,value in count.items():
if val==value:
return key
return "key doesn't exist"
# Getting the max. color as our background
label_background=get_key(max(count.values()))
background_color=ordered_color[label_background]
hex_color_background=rgb2hex(background_color)
#showing our background in the form of an image.
bg=np.zeros((512,512,3),np.uint8)
bg_color=cv2.rectangle(bg,(0,0),(512,512),background_color,-1)
plt.imshow(bg_color)
所有不同顏色的 3D 繪圖
最常見顏色的餅圖
結(jié)論
通過本文,向你展示了兩種技術(shù),你可以在其中使用非常簡單的算法獲取圖像的背景。
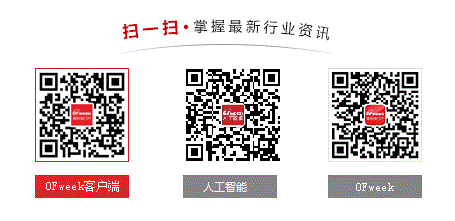
請輸入評論內(nèi)容...
請輸入評論/評論長度6~500個(gè)字
最新活動更多
-
即日-11.13立即報(bào)名>>> 【在線會議】多物理場仿真助跑新能源汽車
-
11月28日立即報(bào)名>>> 2024工程師系列—工業(yè)電子技術(shù)在線會議
-
12月19日立即報(bào)名>> 【線下會議】OFweek 2024(第九屆)物聯(lián)網(wǎng)產(chǎn)業(yè)大會
-
即日-12.26火熱報(bào)名中>> OFweek2024中國智造CIO在線峰會
-
即日-2025.8.1立即下載>> 《2024智能制造產(chǎn)業(yè)高端化、智能化、綠色化發(fā)展藍(lán)皮書》
-
精彩回顧立即查看>> 【限時(shí)免費(fèi)下載】TE暖通空調(diào)系統(tǒng)高效可靠的組件解決方案
推薦專題
- 1 【一周車話】沒有方向盤和踏板的車,你敢坐嗎?
- 2 特斯拉發(fā)布無人駕駛車,還未迎來“Chatgpt時(shí)刻”
- 3 特斯拉股價(jià)大跌15%:Robotaxi離落地還差一個(gè)蘿卜快跑
- 4 馬斯克給的“驚喜”夠嗎?
- 5 打完“價(jià)格戰(zhàn)”,大模型還要比什么?
- 6 馬斯克致敬“國產(chǎn)蘿卜”?
- 7 神經(jīng)網(wǎng)絡(luò),誰是盈利最強(qiáng)企業(yè)?
- 8 比蘋果偉大100倍!真正改寫人類歷史的智能產(chǎn)品降臨
- 9 諾獎(jiǎng)進(jìn)入“AI時(shí)代”,人類何去何從?
- 10 Open AI融資后成萬億獨(dú)角獸,AI人才之爭開啟
- 高級軟件工程師 廣東省/深圳市
- 自動化高級工程師 廣東省/深圳市
- 光器件研發(fā)工程師 福建省/福州市
- 銷售總監(jiān)(光器件) 北京市/海淀區(qū)
- 激光器高級銷售經(jīng)理 上海市/虹口區(qū)
- 光器件物理工程師 北京市/海淀區(qū)
- 激光研發(fā)工程師 北京市/昌平區(qū)
- 技術(shù)專家 廣東省/江門市
- 封裝工程師 北京市/海淀區(qū)
- 結(jié)構(gòu)工程師 廣東省/深圳市